
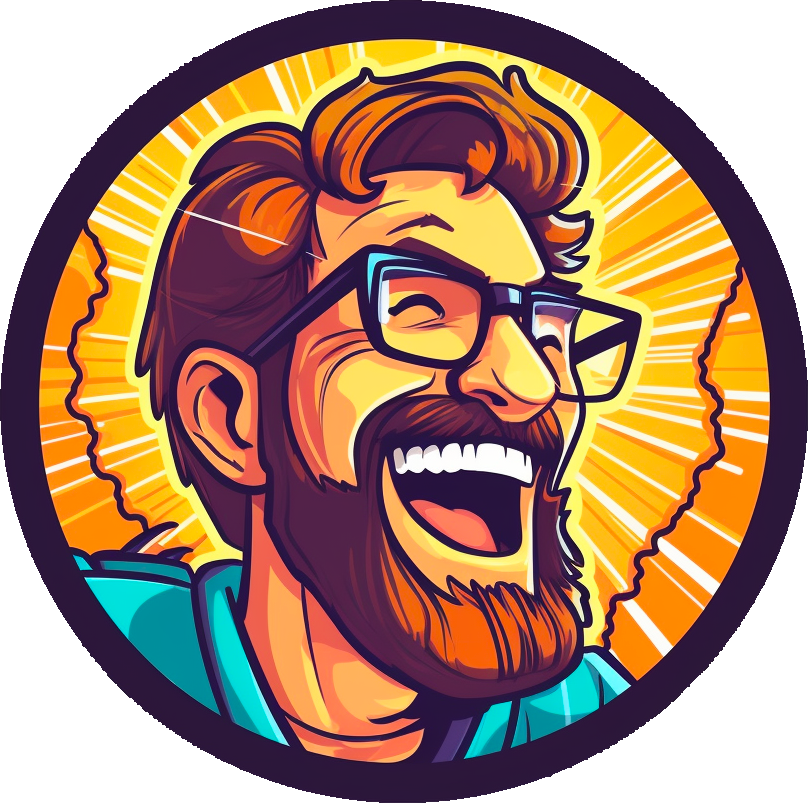
1·
10 hours agoYou already can do that with C++20 concepts and the requires expression
template <typename T>
concept has_member_foo = requires(T t) {
t.foo();
};
// Will fail to instantiate (with nice error
// message) if t.foo() is ill-formed
template <has_member_foo T>
void bar(T t) {
// ...
}
// abbreviated form of above
void baz(has_member_foo auto t) {
// ...
}
// verbose form of above
template <typename T> requires
has_member_foo<T>
void biz(T t) {
// ...
}
// same as above but with anonymous concept
template <typename T> requires
requires(T t) { t.foo(); }
void bom(T t) {
// ...
}
// If already inside a function
if constexpr (has_member_foo<T>) {
// ...
}
// Same but with anonymous concept
if constexpr (requires(T t) { t.foo(); }) {
// ...
}
I’m not sure if there’s anything
enable_if
can do that concepts can’t do somewhat better but yeah there’s definitely a lot of subtleties that reflection is going to make nicer or possible in the first place